1. Overview
This project portfolio serves to document my contributions to CLInic, a CS2103T (Introduction to Software Engineering) project, as part of my Computer Science curriculum in NUS.
CLInic is a desktop application made for clinics. The CLI in CLInic stands for Command Line Interface, which is the main method to use this application. CLInic also has a Graphical User Interface (GUI) to display information in a structured and user-friendly manner. This application uses Java as the primary programming language and uses JavaFX, HTML and CSS for the GUI.
The project scope for CLInic is to morph the given addressbook-level4
into a clinic management application that allows users to manage patient and medicine information and additionally manage the clinic queue.
My task in CLInic is to implement a Patient Queue Management System, as well as to integrate the changes of my team to ensure CLInic works after every enhancement or change.
2. Summary of Contributions
-
Major enhancement: Implementation of Patient Queue Management System (PQMS)
-
What it does: This system allows the clinic to keep track of the queue in the clinic.
-
Justification: This system improves the product significantly because it has created another use case for this application apart from just storing patient and medicine information.
-
Highlights: This system builds upon patient information and medicine information. It models the real life queue system in the clinic and the commands are made to be as intuitive as possible.
-
-
Minor enhancement:
-
Integration of
Patient
andDocument
class with PQMS throughDocumentAddCommand
andDocumentDisplayCommand
-
Integration of
Patient
andMedicine
class throughDispenseMedicineCommand
-
-
Code contributed: [Functional code & Test Code]
-
Other contributions:
3. Contributions to the User Guide
Given below are part of the sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. My full contribution can be viewed here: PQMS Section 3.3 |
Queue Management
You cannot use edit , delete , addmedicalrecord commands on a patient that is in queue. If the patient’s details are changed when
the patient is in queue, the patient will be removed from queue.
|
Registering a patient to Waiting Queue: register
A patient needs to see the doctor? Better get him into the queue using this command!
Alias: reg
Format: register INDEX
Pro tip(s):
Registers the patient at the specified INDEX . The index refers to the index number shown in the displayed patient list. The index must be a positive integer (i.e. 1, 2, 3, …).
|
Example:
-
list
register 3
Registers the 3rd patient in the database.
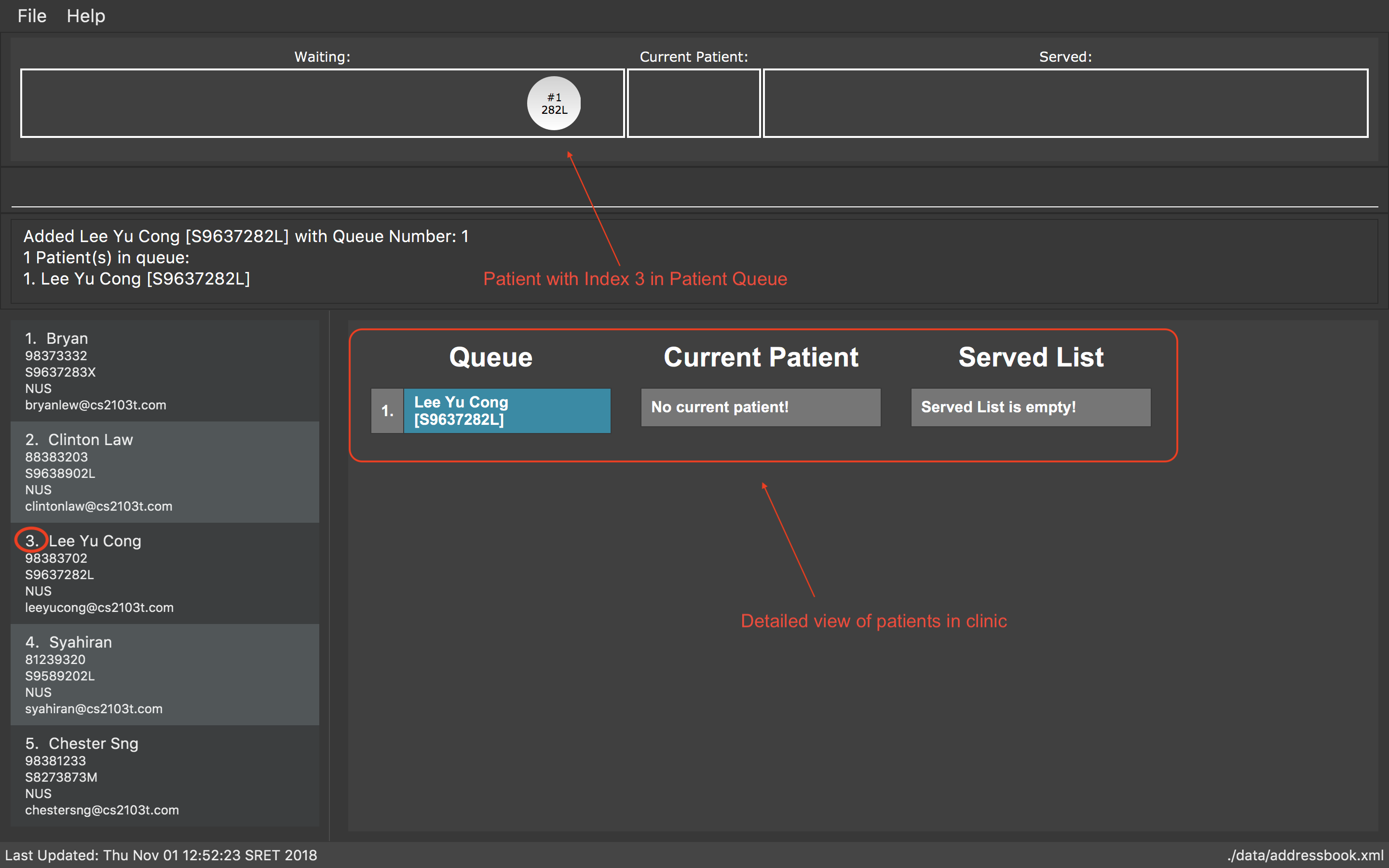
register 3
command-
find david
reg 1
Registers the 1st patient in the resulting list of thefind
command.
Serving the first patient in Waiting queue: serve
Doctor is finally ready to serve the next patient? Use the serve command!
Alias: ser
Format: serve
Pro tip(s):
Upon successful call of this command, the medicine list will be displayed.
|
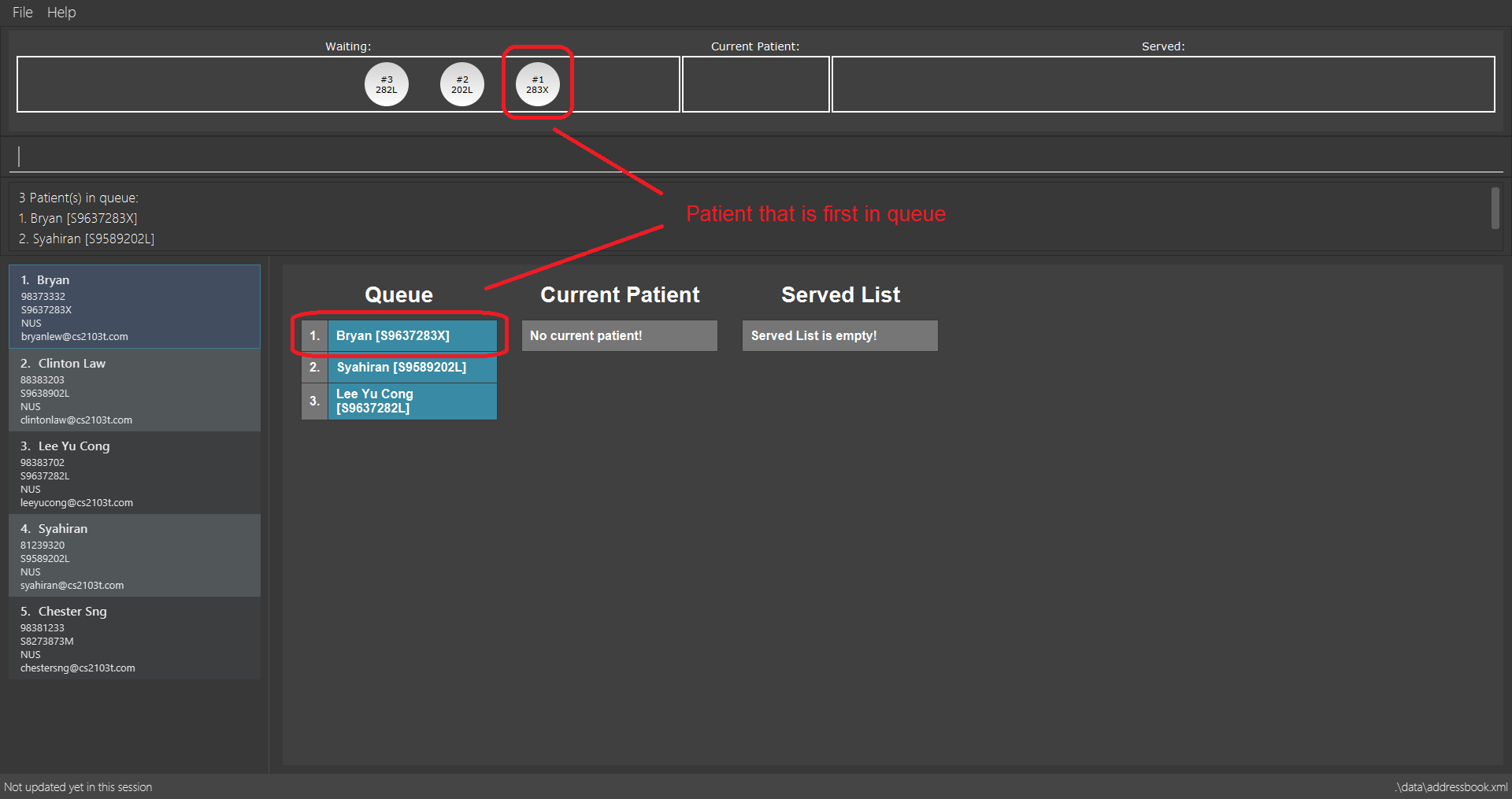
serve
command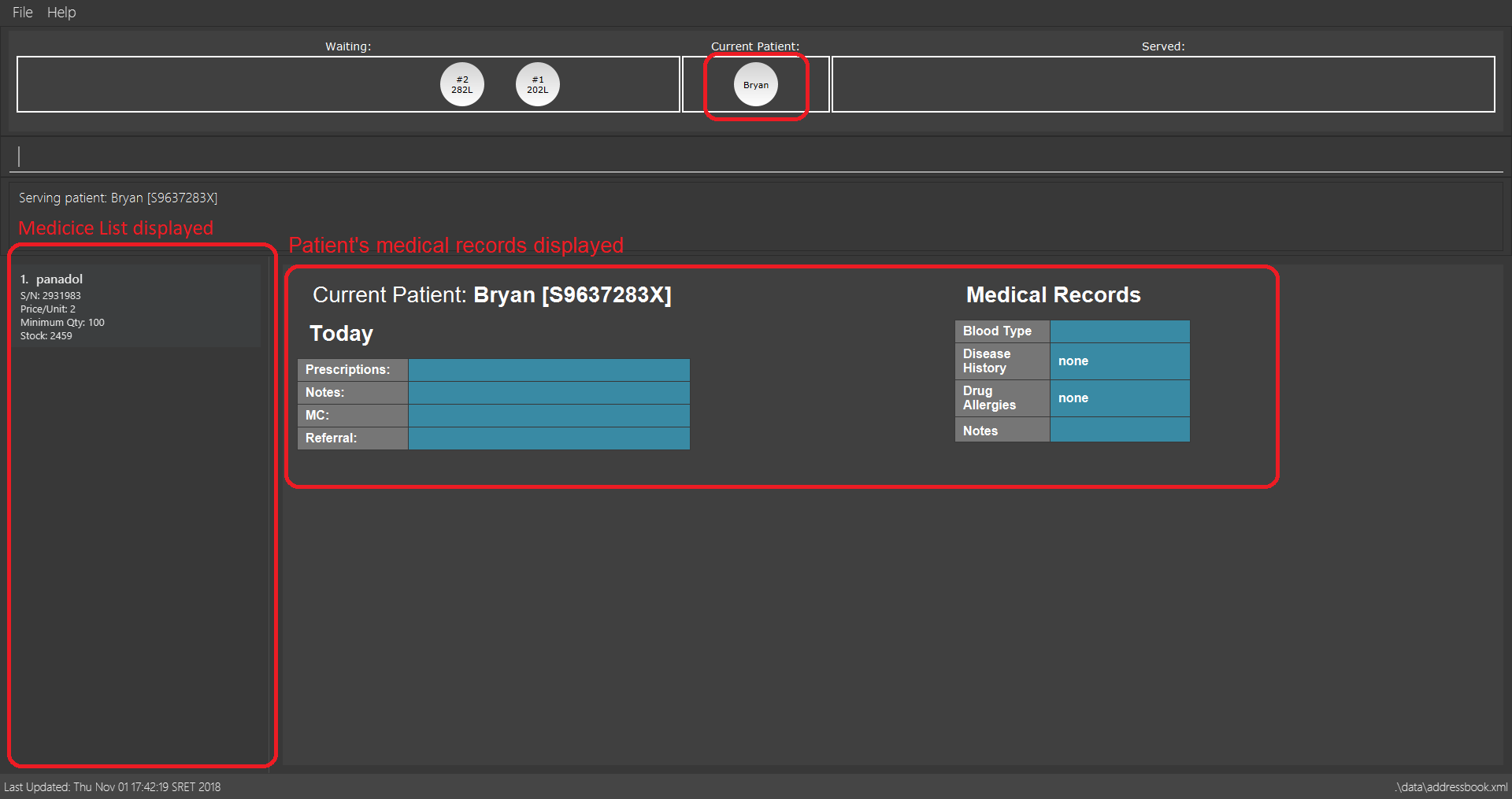
serve
commandAdding document contents to Current Patient: adddocument
Patient has a bad headache? Patient needs 5 days mc? Better write them down!
Alias: ad
Format: adddocument [mc/MC_DAYS] [n/NOTES] [r/REFERRAL]
Pro tip(s):
|
Examples:
-
adddocument mc/3 n/Headache for the past 2 days
Adds MC duration and notes to the Current Patient.
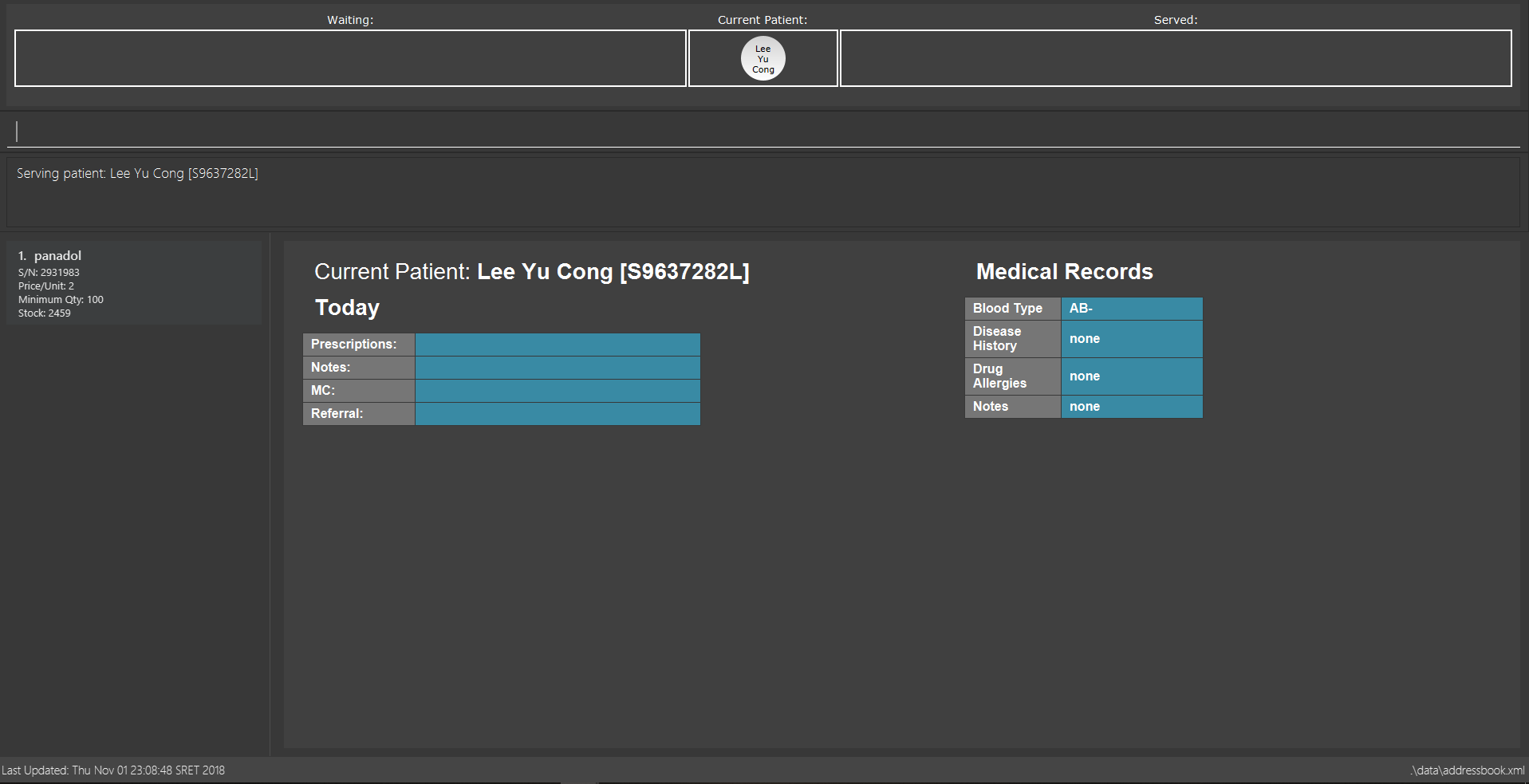
adddocument mc/3 n/Headache for the past 2 days
command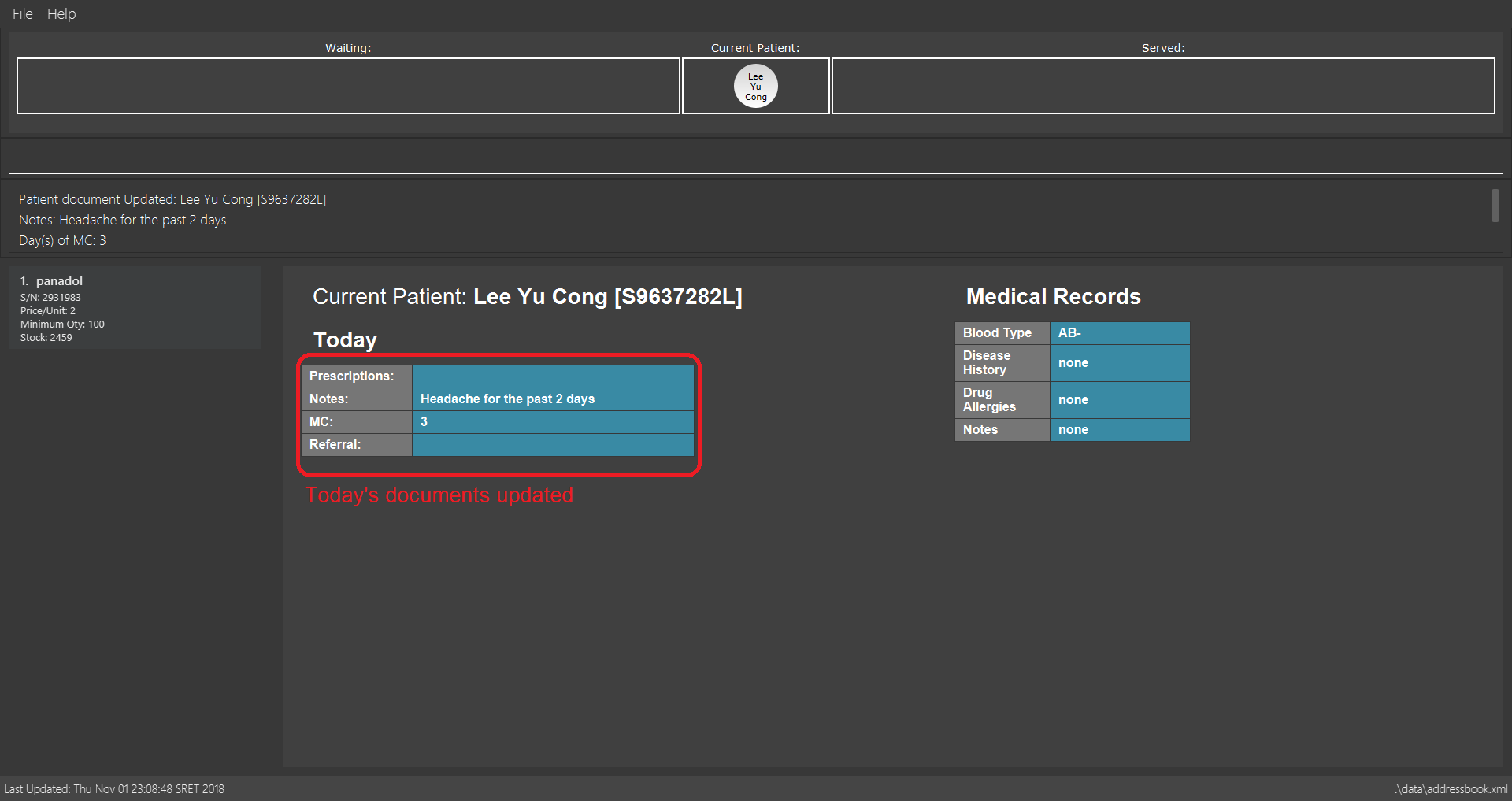
adddocument mc/3 n/Headache for the past 2 days
command-
ad r/Ng Teng Fong Hospital
Adds referral to the Current Patient.
4. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Patient Queue Management System
The Patient Queue Management System (PQMS) consists of three main classes: PatientQueue
, CurrentPatient
and ServedPatientQueue
. A patient that
registers to see the doctor is put into the PatientQueue
. When it is the patient’s turn to see the doctor, he will become the CurrentPatient
. After
he is done with seeing the doctor, he will become a ServedPatient
and put into the ServedPatientQueue
to await payment and document processing.
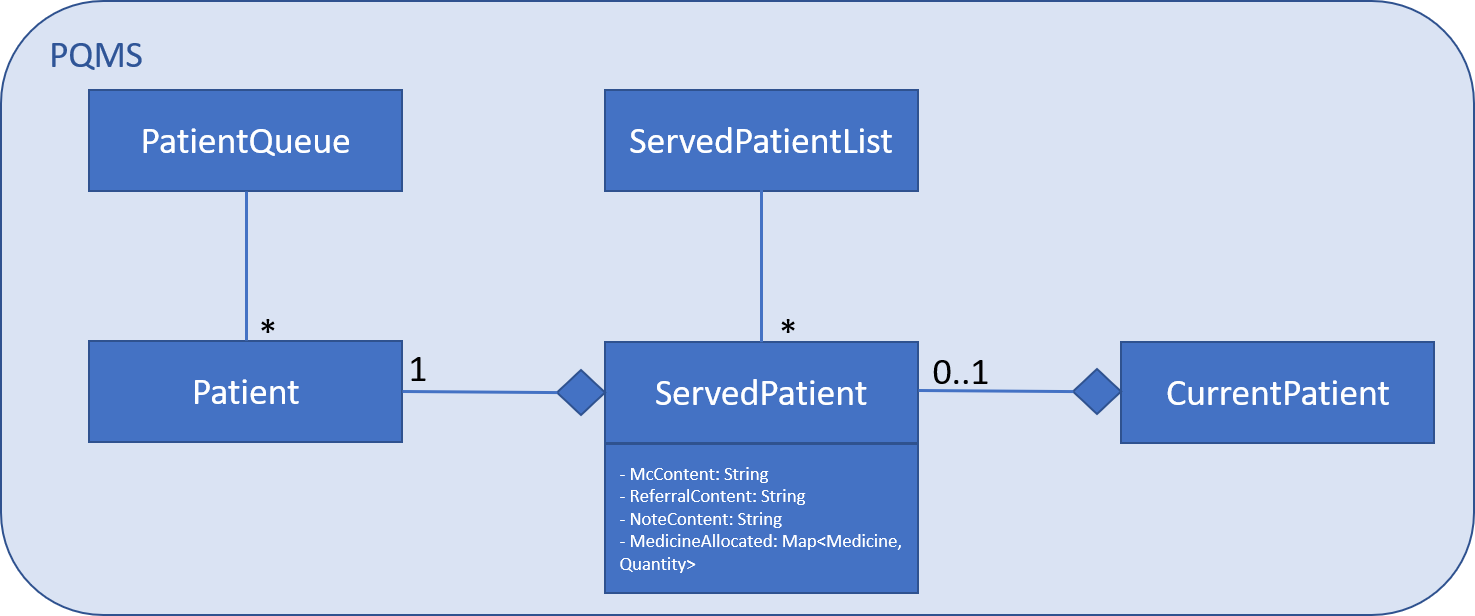
ServedPatient
acts as a wrapper for Patient
, storing intermediate information that are likely to change during the patient’s visit to the clinic.
CurrentPatient
acts as a wrapper for ServedPatient
, updating the intermediate information that it contains. These intermediate information are
McContent
, ReferralContent
, NoteContent
and Medicine
allocated.
The PQMS consists of the register
, insert
, remove
, serve
, adddocument
, displaydocuments
, dispensemedicine
, finish
, payment
and Document
commands.
These commands inherit from QueueCommand
because it will require more arguments in its execute command. The PQMS is not inside Model
as it is only required during runtime;
it does not need to be saved into the database. A new QueueCommand
abstract class that inherits from Command
is created and it defines a new method signature of execute
.
The following code snippet shows how the QueueCommand
is implemented:
public abstract class QueueCommand extends Command {
public CommandResult execute(Model model, CommandHistory history) throws CommandException {
throw new CommandException(Messages.MESSAGE_WRONG_EXECUTE_COMMAND);
}
public abstract CommandResult execute(Model model, PatientQueue patientQueue, CurrentPatient currentPatient,
ServedPatientList servedPatientList, CommandHistory history) throws CommandException;
}
The follow code snippet shows how the RegisterCommand
makes use of both the Model
and PatientQueue
of the PQMS.
public CommandResult execute(Model model, PatientQueue patientQueue, CurrentPatient currentPatient,
ServedPatientList servedPatientList, CommandHistory history) throws CommandException {
requireNonNull(patientQueue);
List<Patient> lastShownList = model.getFilteredPersonList();
if (targetIndex.getZeroBased() >= lastShownList.size()) {
throw new CommandException(Messages.MESSAGE_INVALID_PERSON_DISPLAYED_INDEX);
}
Patient patientToRegister = lastShownList.get(targetIndex.getZeroBased());
if (patientQueue.contains(patientToRegister) || currentPatient.isPatient(patientToRegister)
|| servedPatientList.containsPatient(patientToRegister)) {
throw new CommandException(MESSAGE_DUPLICATE_PATIENT);
}
int position = patientQueue.enqueue(patientToRegister);
return new CommandResult(MESSAGE_SUCCESS + patientToRegister.toNameAndIc()
+ " with Queue Number: " + position + "\n" + patientQueue.displayQueue());
}
For better illustration, the following sequence diagram shows how the register
commands works:
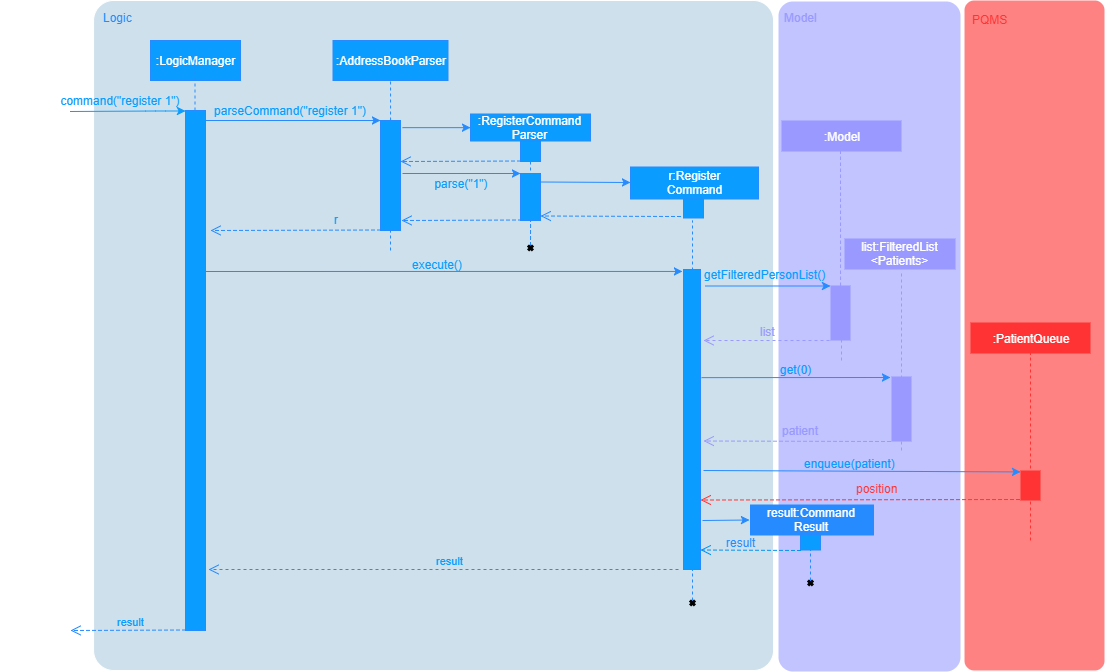
The other PQMS commands roughly follow the same sequence as Register
command but can interact with CurrentPatient
or ServedPatientList
instead of PatientQueue
.
Given below is an example usage scenario of the PQMS.
Step 1. Three patients visits the clinic, the user executes the register
command for each patient. If these patients are not registered in CLInic’s database they need to be added first.
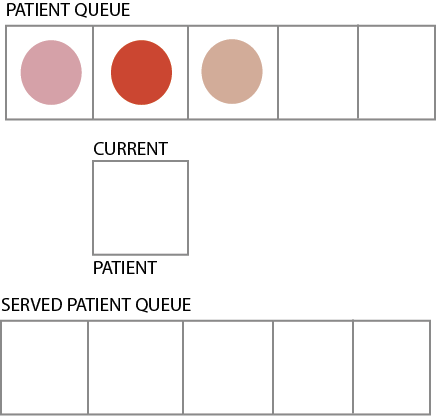
Step 2. The doctor is ready for the next patient. The user executes the serve
command to serve the first patient in the queue.
The patient is now the CurrentPatient
.
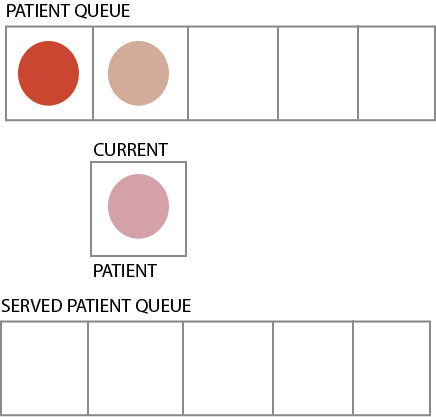
Step 3. The user executes the adddocument
command to add information to the CurrentPatient
. These information will be used for document
processing later on.
Step 4. The CurrentPatient
is done with the consultation. The user executes the finish
command to transfer this patient to
the ServedPatientQueue
. Afterwards, the user executes serve
to serve the next patient in PatientQueue
.
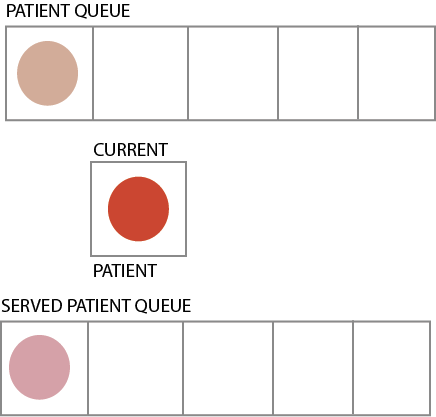
Step 5. The user executes various Document
commands to generate necessary documents for the served patient.
Step 6. The user finally executes payment
command to end the patient’s visit to the clinic, removing
him from the ServedPatientQueue
.
Design Considerations
Aspect: Proper OOP practice
Relationship between CurrentPatient
, ServedPatient
and Patient
.
-
Current Implementation: Composition
-
CurrentPatient
is composed ofServedPatient
.ServedPatient
is composed ofPatient
. -
Pros: Easy manipulation of composed class.
-
Cons: Weird relationship between the three classes. i.e.
ServedPatient
has-aPatient
.
-
-
Alternative: Inheritance
-
CurrentPatient
inherits fromServedPatient
.ServedPatient
inherits fromPatient
.-
Pros: Proper relationship between three classes.
-
Cons: Cannot cast
Patient
toServedPatient
orCurrentPatient
for usage in PQMS.
-