Overview
Hello, I am Syahiran. As part of my undergraduate study of Computer Science (CS) at the National University of Singapore (NUS), I undertook a Software Engineering module (CS2103T) in my third year. Together with four other students from CS, we modified and improved the given address book application into a database management system called CLInic. This project portfolio serves to document my contributions to this project.
CLInic is a desktop application, which means it can run on a computer’s operating system without the need for a web browser. It is designed specifically for clinics, and helps to reduce the manual labour of daily administrative tasks like:
-
Maintaining a database of patients and their medical records
-
Checking and updating medicine inventory
-
Generating receipts, medical certificates and referral letters for patients
CLI is an acronym for "Command Line Interface", as CLInic works by typing commands directly into the application through the CLI. Together with CLInic’s clean Graphical User Interface (GUI), the application allows for an easy and intuitive experience for both technical and non-technical users alike.
Summary of Contributions
This section summarises my contributions to the project, and provides links to my code and pull requests. |
-
Major enhancement: I supported the implementation of the
Document
class, along with its accompanying extending classesMedicalCertificate
andReferralLetter
.-
What it does: This feature allows for the doctor to input information, like the duration of medical leave and referred location, to easily generate medical certificate and referral letter documents in the form of HTML files.
-
Justification: This feature takes in the relevant information that has been keyed in and structures them in a way that is presentable and professional. This can help to reduce the incidences of error that arise due to illegible handwriting.
-
Highlights: This feature is intended to decrease the need for pen and paper documentation. Most people can type significantly faster than they can write. Also, legibility of handwriting may affect comprehension. In the case of referral letters, where vital medical information may be conveyed, we want the information to be presented as clearly as possible.
-
-
Minor enhancement: I added shorthand codes for most of the commands in CLInic to reduce the amount of typing necessary when using the CLI.
-
Justification: Some commands like
dispenseMedicine
andMedicalCertificate
are really long, so it can be troublesome for the user to type the whole command in order to execute the command. Instead, users can typedm
andmc
to execute the same respective commands.
-
-
Code contributed: View my code for the project here!
-
Other contributions:
Contributions to the User Guide
Provided below are excerpts of my contributions to the User Guide. This section showcases my ability to write documentation targeting end-users. |
Generating medical certificate: mc
Does the patient need to be excused from school or work to rest? Let’s give him a medical certificate!
Format: mc INDEX
The patient’s INDEX in the Served Patient Queue must be a positive integer (i.e. 1, 2, 3, …).
|
Examples:
-
mc 1
Generates a medical certificate for the 1st patient in the Served Patient Queue.
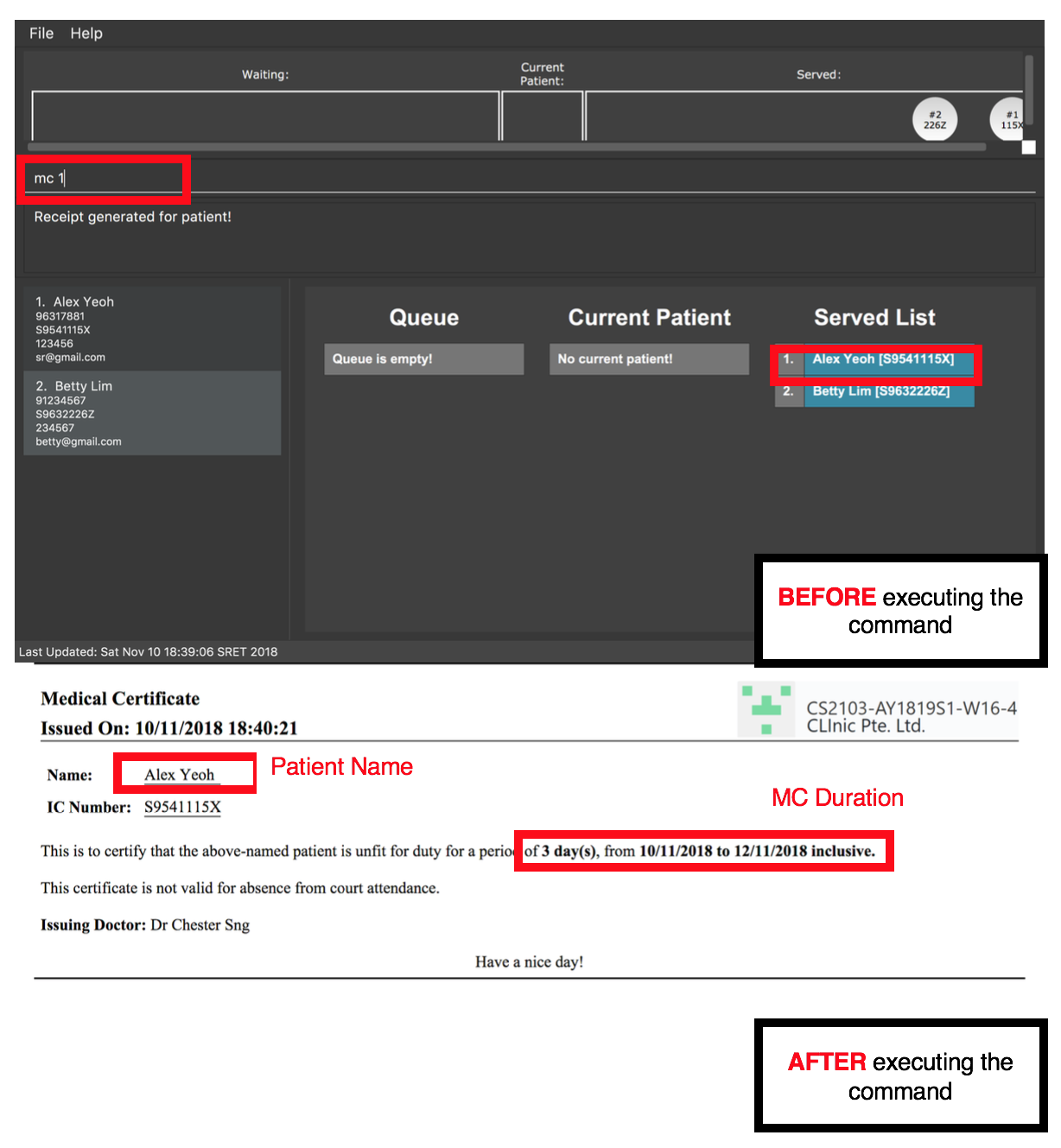
Alex Yeoh
.Generating referral letter: refer
Does the patient need to consult a specialist? Let’s give him a referral letter!
Alias: ref
Format: refer INDEX
The patient’s INDEX in the Served Patient Queue must be a positive integer (i.e. 1, 2, 3, …).
|
Examples:
-
refer 7
Generates a referral letter for the 7th patient in the Served Patient Queue.
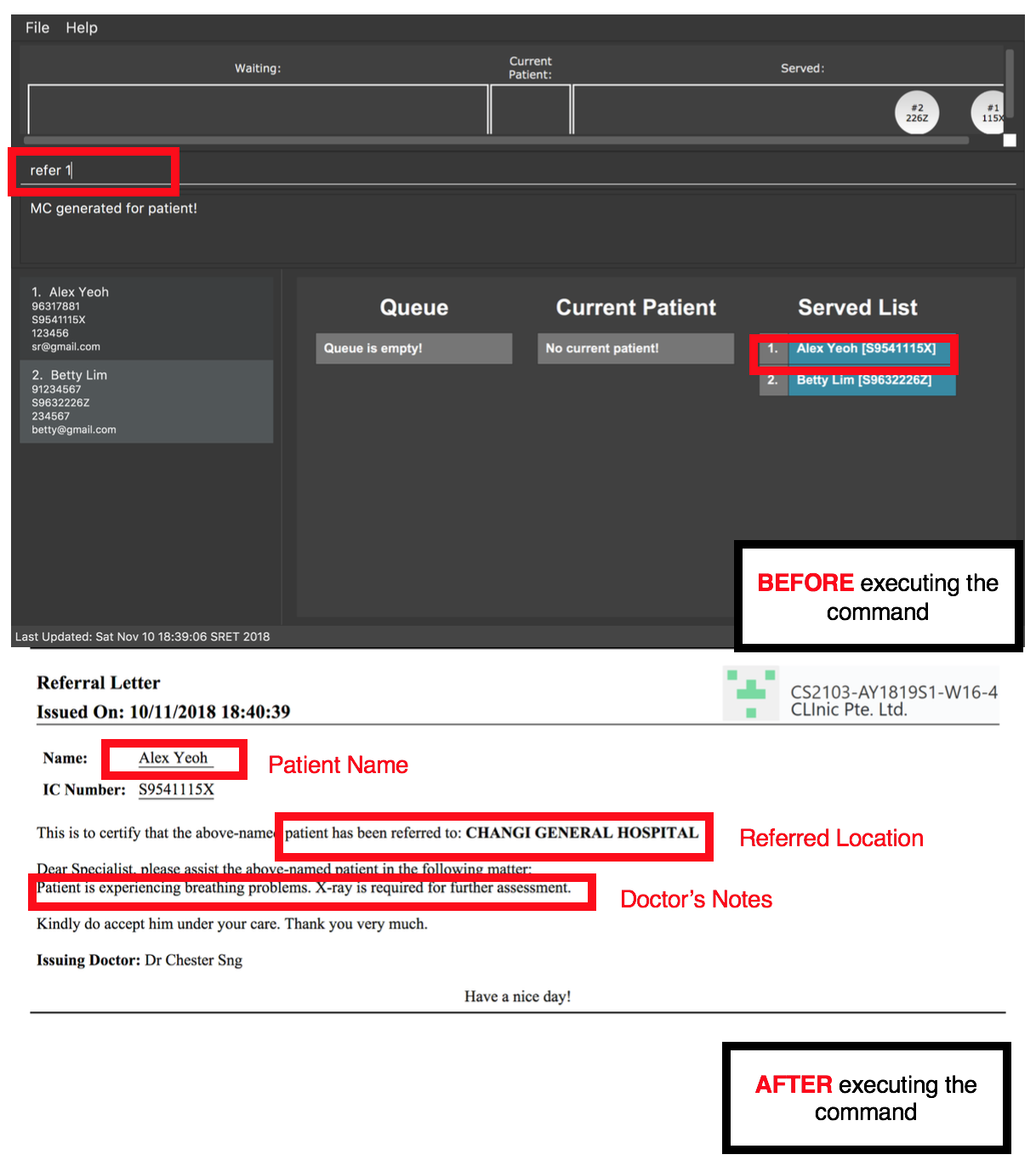
Alex Yeoh
.Command Summary
Patient
Command Word | Command Alias | Format | Example |
---|---|---|---|
add |
|
|
|
list |
|
|
|
edit |
|
|
|
find |
|
|
|
delete |
|
|
|
select |
|
|
|
addmedicalrecord |
|
|
|
Medicine
Command Word | Command Alias | Format | Example |
---|---|---|---|
addmedicine |
|
|
|
editmedicine |
|
|
|
restock |
|
|
|
liststock |
|
|
|
findmedicine |
|
|
|
checkstock |
|
|
|
Contributions to the Developer Guide
Provided below are excerpts of my contributions to the Developer Guide. This section showcases my ability to write technical documentation and the technical depth of my contributions to the project. |
Implementation of MedicalCertificate
The MedicalCertificate
class structure is as follows:
-
Has attributes of the
ServedPatient
it is generated from, which includes theServedPatient
'sname
andIcNumber
.-
These attributes are marked as
final
as they should not be changed.
-
-
Contains additional information like the duration, start-date and end-date of their medical leave.
Users can generate a MedicalCertificate
for a specific ServedPatient
by invoking the MedicalCertificateCommand
accompanied by the ServedPatient
's index in the ServedPatientList
.
The below sequence diagram illustrates how the MedicalCertificateCommand
works.
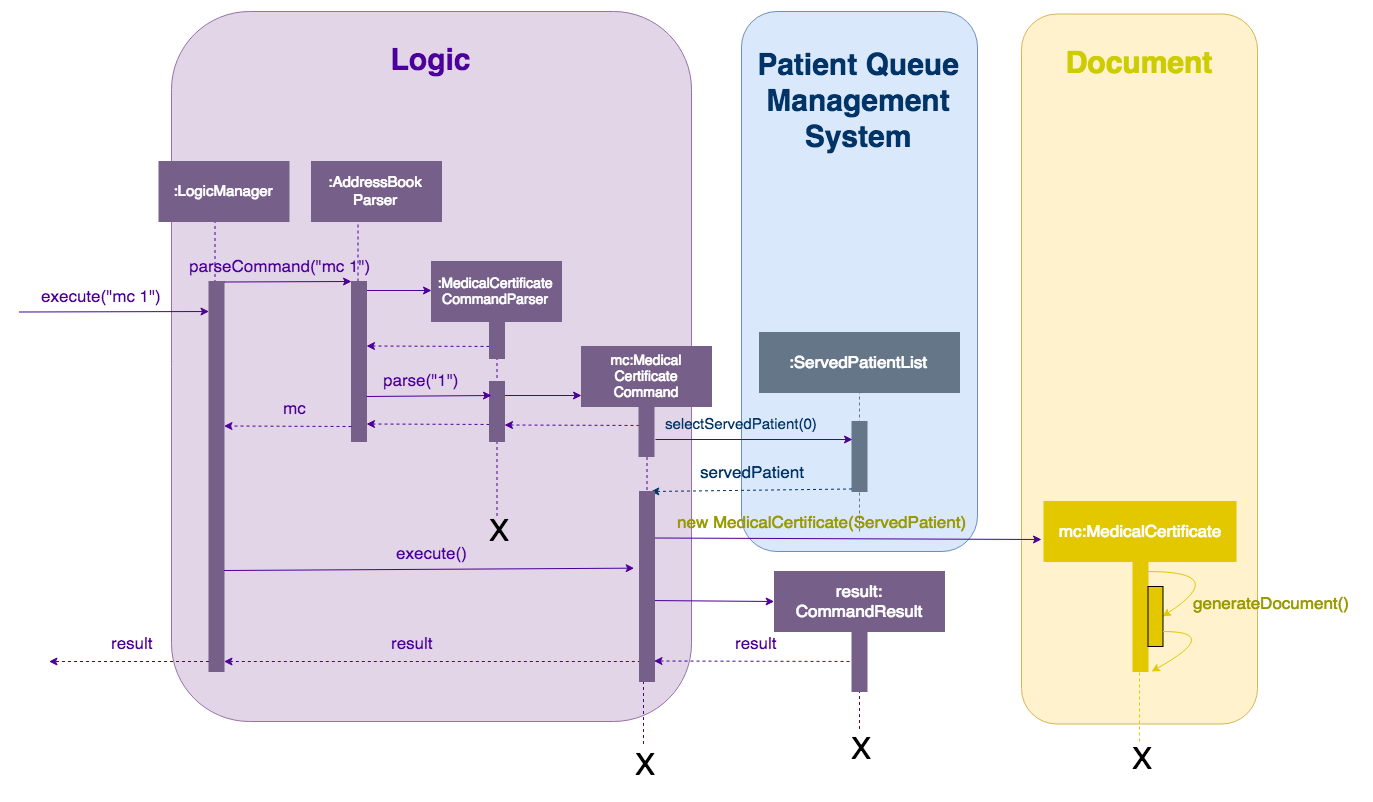
When the MedicalCertificateCommand
is executed, it will construct a new MedicalCertificate
object and extract the relevant information
from the ServedPatient
specified by the index
.
Shown below is how information like numMcDays
is formatted into the medical certificate document template.
public String formatInformation() {
int numMcDays = getMcDays();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("This is to certify that the above-named patient is unfit for duty for a period of ")
.append("<b>" + numMcDays + "</b>")
.append(" <b>day(s)</b>, from ")
.append("<b>" + LocalDate.now().format(formatter) + "</b>")
.append(" <b>to</b> ")
.append("<b>" + LocalDate.now().plusDays(numMcDays - 1).format(formatter) + "</b>")
.append(" <b>inclusive.</b><br><br>")
.append("This certificate is not valid for absence from court attendance.<br><br>")
.append("<b>Issuing Doctor:</b> Dr Chester Sng" + "<br>");
return stringBuilder.toString();
}
Executing mc with an invalid index will not result in the generation of a MedicalCertificate .
|
Implementation of ReferralLetter
The ReferralLetter
class structure is as follows:
-
Has attributes of the
ServedPatient
it is generated from, which includes theServedPatient
'sname
andIcNumber
.-
These attributes are marked as
final
as they should not be changed.
-
-
Contains additional information like the doctor’s notes and patient’s referred location.
Users can generate a ReferralLetter
for a specific ServedPatient
by invoking the ReferralLetterCommand
accompanied by the ServedPatient
's index in the ServedPatientList
.
The below sequence diagram illustrates how the ReferralLetterCommand
works.
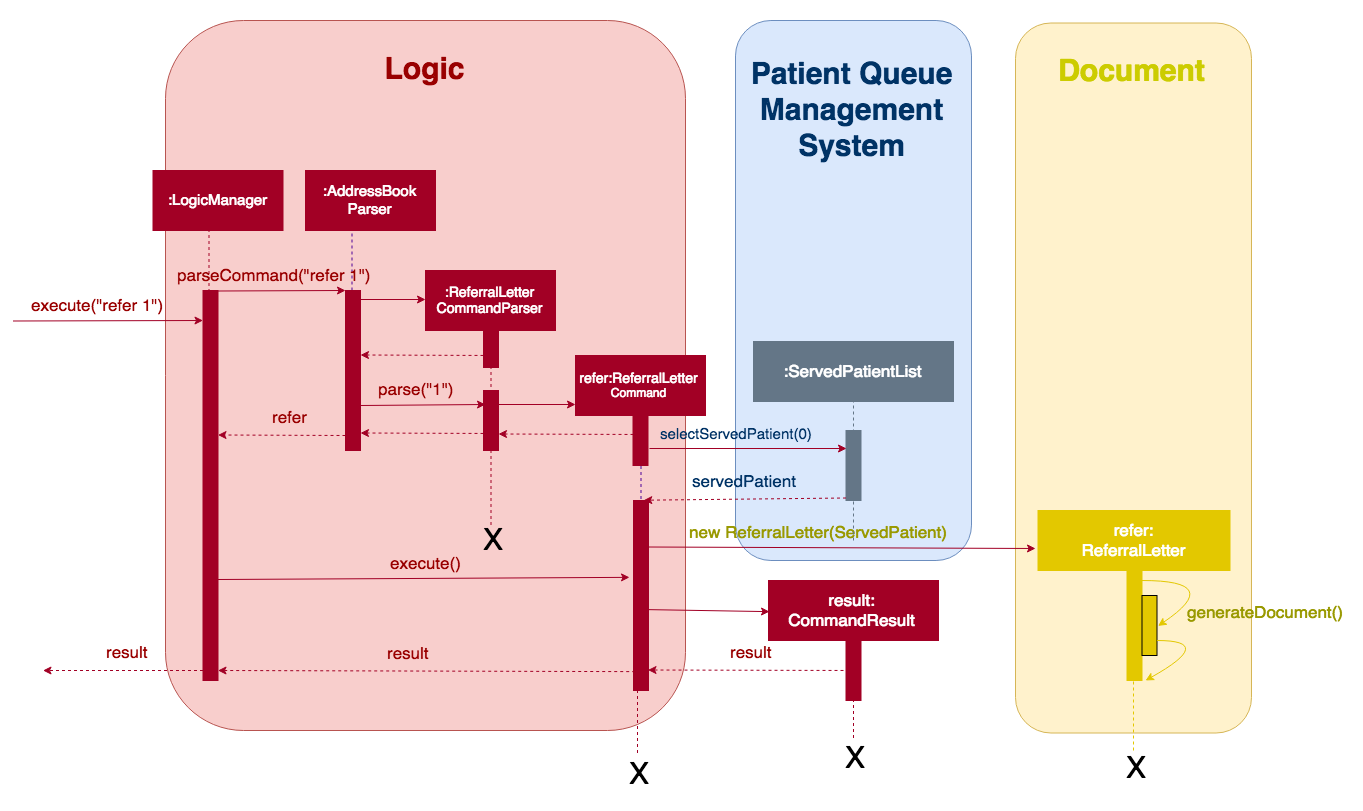
When the ReferralLetterCommand
is executed, it will construct a new ReferralLetter
object and extract the relevant information
from the ServedPatient
specified by the index
.
Shown below is how information like referralContent
and noteContent
are formatted into the referral letter document template.
public String formatInformation() {
String referralContent = getReferralContent();
String noteContent = getNoteContent();
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("This is to certify that the above-named patient has been referred to: ")
.append("<b>" + referralContent.toUpperCase() + "</b>" + "<br><br>")
.append("Dear Specialist, please assist the above-named patient in the following matter:<br>")
.append(noteContent + "<br><br>")
.append("Kindly do accept him under your care. Thank you very much.<br><br>")
.append("<b>Issuing Doctor:</b> Dr Chester Sng" + "<br>");
return stringBuilder.toString();
}
Executing refer with an invalid index will not result in the generation of a ReferralLetter .
|
Design Considerations
Aspect: Implementation of adddocument
, MedicalCertificate
and ReferralLetter
Commands
-
Alternative 1 (current choice): The issuing doctor’s name is hard-coded into the
MedicalCertificate
andReferralLetter
HTML template.-
Pros: No need for an extra parameter to key in the issuing doctor’s name, which is helpful when there is only one doctor working in a clinic.
-
Cons: There may be more than one doctor working in a clinic.
-
-
Alternative 2: Include a mandatory parameter
id/ISSUING_DOCTOR
to theadddocument
command for the issuing doctor to key in his/her name.-
Pros: Different doctors can sign off the medical certificates and referral letters.
-
Cons: Slightly more typing is necessary before doctors can execute the
adddocument
command.
-