1. Introduction
Hello, I am Yu Cong. I am currently studying Computer Science as my major in School of Computing, National University of Singapore. I formed a team with 4 other students to create CLInic for the CS2103T module (Introduction to Software Engineering) as part of my university education.
This project portfolio serves to document my contributions to this project. You can see my contributions to the project, user guide and developer guide below.
CLInic is a desktop application designed for clinics who wish to automate their daily operations.
CLI in CLInic is an acronym for Command Line Interface, which is the primary method to use the application.
Unlike traditional address books, the doctor or clinic receptionist can easily add
, edit
or find
the information of
patient or medicine by entering just one simple line in the command box.
This is what CLInic has to offer:
-
Efficient management of patient and medicine information.
-
Logical system to control the patient queue.
-
Customised receipts, medical certificates and referral letters for clinics.
Want to make your clinic more organised? Download CLInic and try it now for FREE!
2. Summary of Contributions
In this section, you can find the major and minor enhancement that I made for CLInic, as well as my contributions to the project.
-
Major enhancement: Implementation of medicine records system in CLInic.
-
What it does: It allows the clinic receptionist to automate the tracking of the amount of medicines.
-
Justification: The amount of medicines is tracked in the application instead of paper and pen. This reduces the human error made by messy administrative work or negligence. There are also useful commands that can organise or find the information of a medicine. With this, you do not have to manually note down the change in level of medicine every time a medicine is dispensed.
-
Highlights: With the
checkstock
command, you can easily list out all medical stocks that are low in stock in just 2 seconds!
-
-
Minor enhancement:
-
Rephrased output messages to make it more comprehensible.
-
-
Code contributed:
-
Click here to see what I wrote!
-
-
Other contributions:
-
Wrote the test utilities for other team members to build their tests upon.
-
Advised my team to apply knowledge learned in lecture where necessary.
-
Configured config.json to allow Reposense to detect each member’s works.
-
Redrew the Structure of the Model Component in the Developer Guide to model CLInic better.
-
3. Contributions to the User Guide
Given below are the sections that I have contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Medicine Records System
Adding a medicine: addmedicine
Bought the latest medicine that effectively cures eczema?
Use addmedicine
to add that medicine into CLInic now!
Alias: am
Format: addmedicine mn/MEDICINE_NAME msq/MINIMUM_STOCK_QUANTITY ppu/PRICE_PER_UNIT sn/SERIAL_NUMBER s/STOCK
|
Example:
-
addmedicine mn/panadol msq/500 ppu/0.50 sn/91853 s/1000
Adds a medicine namedpanadol
with minimum stock quantity of500
units, price per unit of$0.50
, serial number of91853
and stock of1000
units to the CLInic inventory. -
am mn/asprin msq/100 ppu/0.20 sn/53068 s/100
Adds a medicine namedasprin
with minimum stock quantity of100
units, price per unit of$0.20
, serial number of53068
and stock of100
units to the CLInic inventory.
See below for a pictorial guide on how to add aspirin to the records:
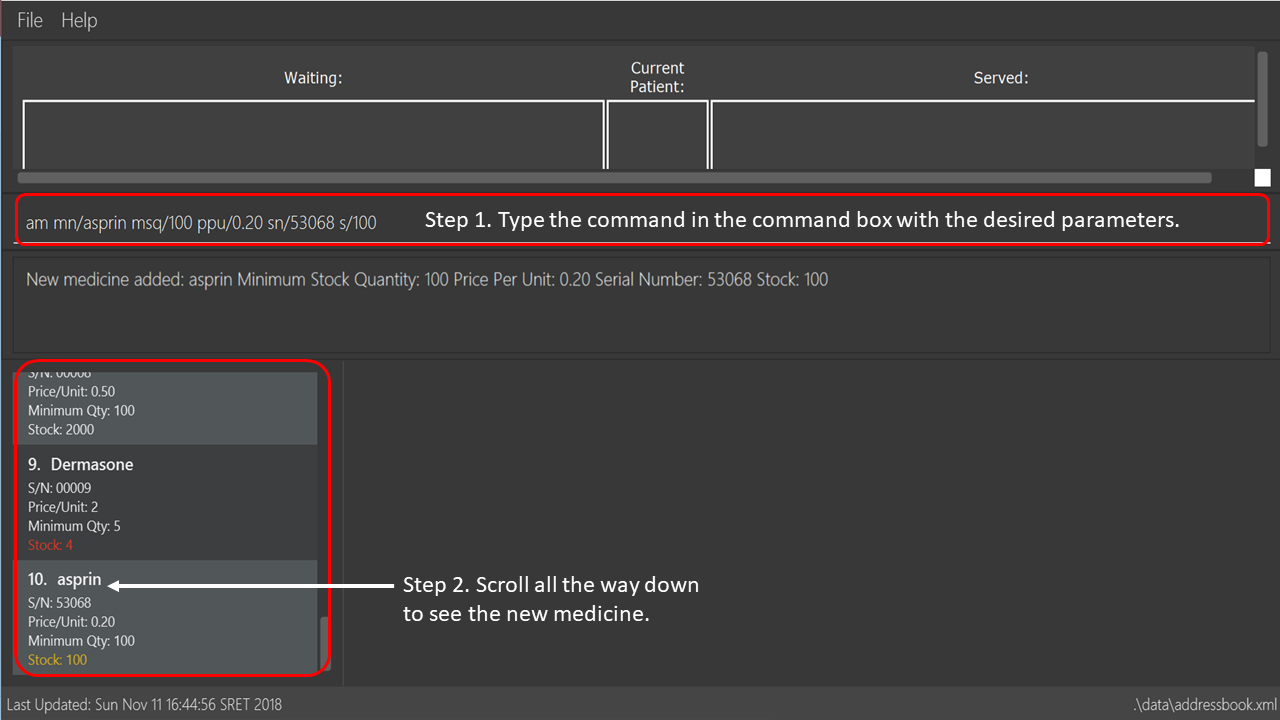
addmedicine
commandFinding details of a medicine: findmedicine
Need the price of a medicine urgently?
Use findmedicine
to search for
the details of a medicine from its medicine name!
Alias: fm
Format: findmedicine MEDICINE_NAME
You can enter more than one name to get the results of all medicines with the names entered. Refer to the third example below. |
Example:
-
findmedicine panadol
Finds the details of the medicines with the name panadol. -
fm chlorpheniramine
Finds the details of the medicines with the name chlorpheniramine. -
fm telfast panadol
Finds the details of all medicines with the name telfast or panadol.
See below for a pictorial guide on how to find telfast or panadol in CLInic:
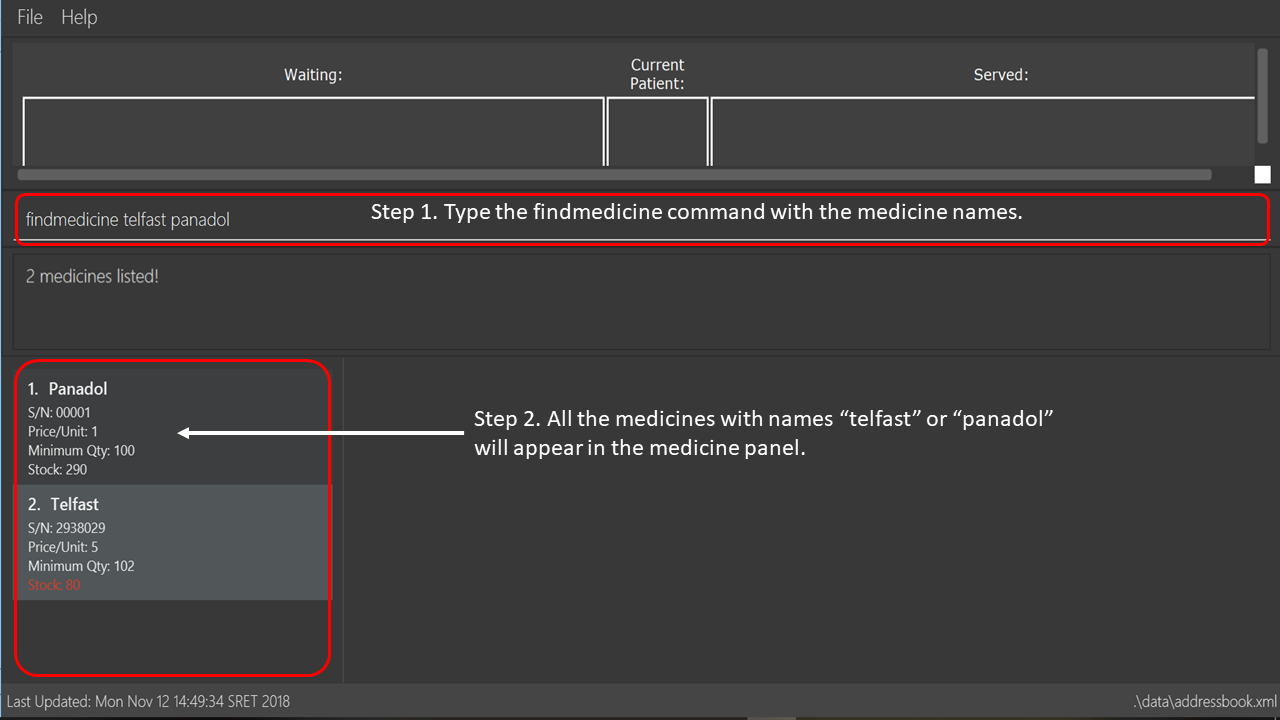
findmedicine
commandListing medicines that are low in supply: checkstock
No time to do stock taking?
Use checkstock
and get it done in under 2 seconds!
Alias: cs
Format: checkstock
Medicines that are low in supply are defined as those whose stock levels are lower than or equal to their minimum stock quantities. |
See below for a pictorial guide on how to use checkstock
:
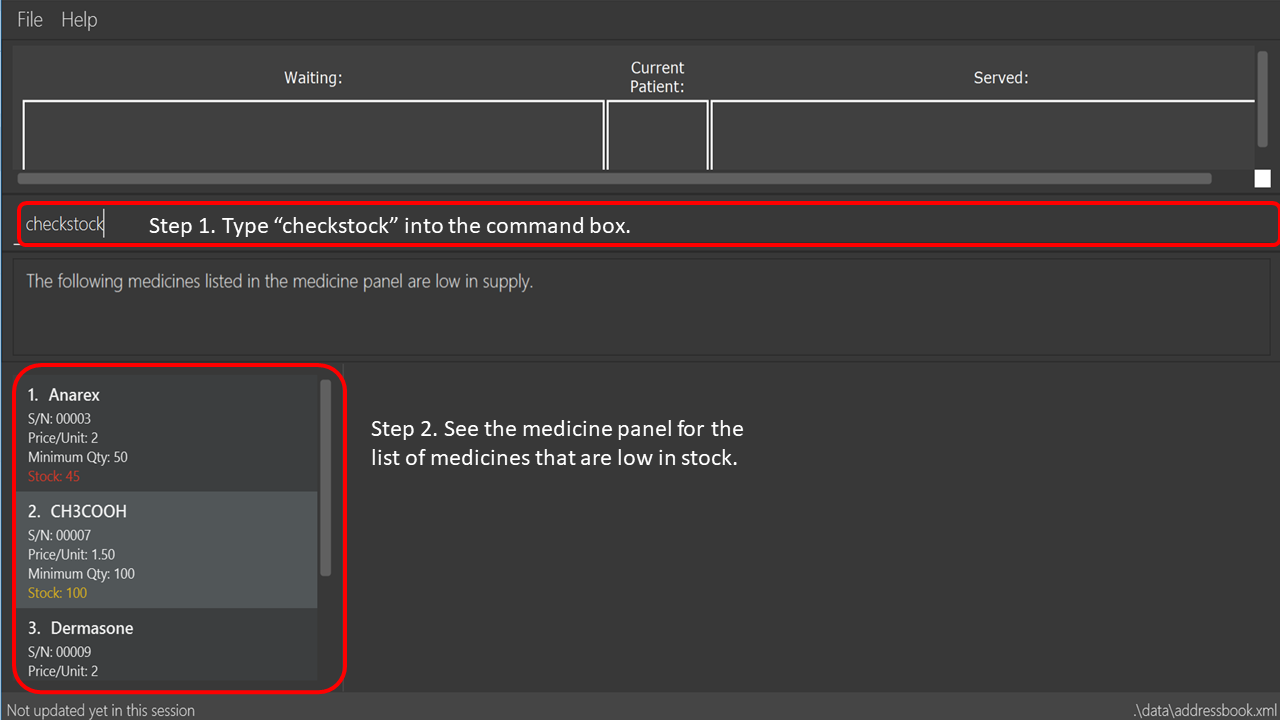
checkstock
command4. Contributions to the Developer Guide
Given below are the sections that I have contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Medicine Records System
The medicine records system is used to manage the medicine inventory in the clinic. It allows the clinic to automate the monitoring and management of their medicine supply easily. There is great potential for this system to evolve, for example:
-
Using artificial intelligence to manage the clinic supply without human intervention.
-
Delivering first-hand information from medical breakthroughs to treat patients more effectively.
Current Implementation
The Medicine class and the properties of the medicine are located in Model. They are modeled after the properties of a real medicine in a clinic context. The list of medicines currently tracked in the clinic is located in the AddressBook class.
Refer to the diagram below for the commands that involves the Medicine Class:
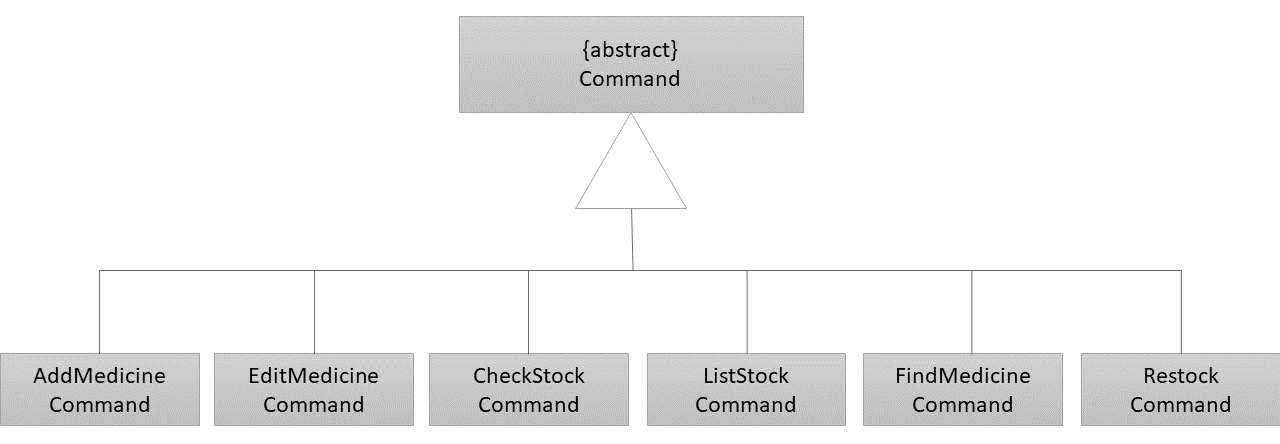
Given below are examples of how the medicine commands are used:
Scenario 1: The clinic orders a new medicine that they do not currently possess.
The clinic receptionist uses the addMedicine
command to add the new medicine into the records.
Scenario 2: There is a wrong entry for one of the serial number of the medicine. The user uses the
editmedicine
command to correct the serial number. The code on how it works is shown below:
public CommandResult execute(Model model, CommandHistory history) throws CommandException {
requireNonNull(model);
List<Medicine> lastShownList = model.getFilteredMedicineList();
if (index.getZeroBased() >= lastShownList.size()) {
throw new CommandException(Messages.MESSAGE_INVALID_MEDICINE_DISPLAYED_INDEX);
}
Medicine medicineToEdit = lastShownList.get(index.getZeroBased());
Medicine editedMedicine = createEditedMedicine(medicineToEdit, medicineDescriptor);
if (!medicineToEdit.isSameMedicine(editedMedicine)
&& model.hasMedicine(editedMedicine)) {
throw new CommandException(MESSAGE_DUPLICATE_MEDICINE);
}
/* The following three methods checks if the new medicine name or
new serial number that is entered used by other medicines in CLInic.
If so, they throw an error specifying which of the two is duplicated.
This is done as the medicine name and serial number are unique to a medicine.
*/
checkBothNewMedicineNameAndSerialNumberAlreadyExisting(model, editedMedicine);
checkNewSerialNumberAlreadyExisting(model, editedMedicine);
checkNewMedicineNameAlreadyExisting(model, editedMedicine);
model.updateMedicine(medicineToEdit, editedMedicine);
model.updateFilteredMedicineList(Model.PREDICATE_SHOW_ALL_MEDICINES);
model.commitAddressBook();
EventsCenter.getInstance().post(new ShowMedicineListEvent());
return new CommandResult(String.format(MESSAGE_EDIT_MEDICINE_SUCCESS, editedMedicine));
}
}
Scenario 3: The doctor prescribes the patient he is serving a medicine. The dispensemedicine
command is entered.
The stock level of the medicine is then updated. The sequence diagram below shows how the code works:
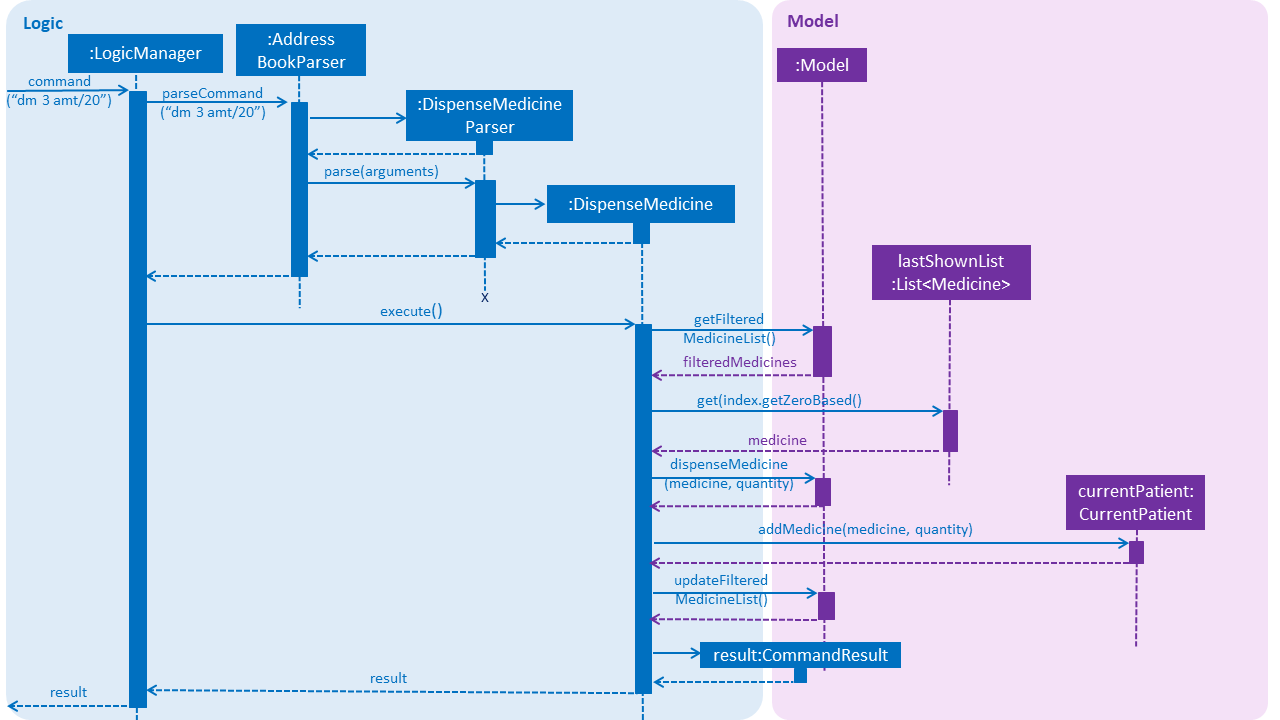
dispensemedicine
Scenario 4: The clinic receptionist needs to check for the stock level of every medicine.
He executes the checkStock
command
which lists all medicines that are below their Minimum Stock Quantity. See the figure below for an example
of how the filter works:
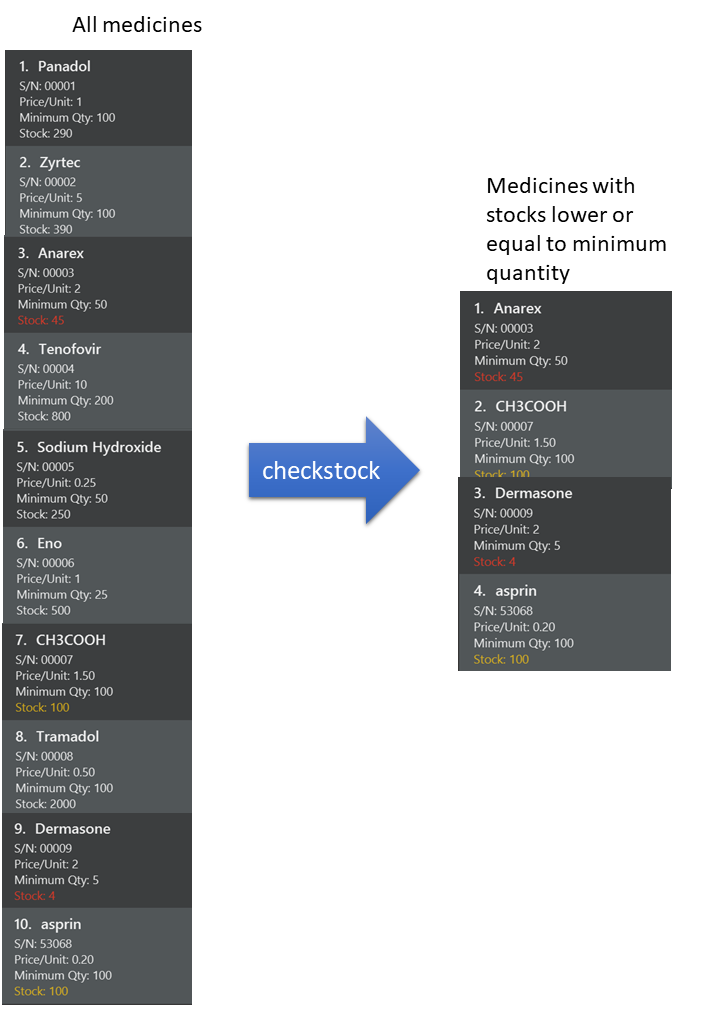
Design Considerations
The medicines in the clinic need to be stored in a Java Collection. We considered using a list or a hash map. See below for the analysis of each choice:
Aspect: Execution of the command
-
Current choice: Stores the medicines in a list.
-
Pros: Many easy and useful methods to manipulate medicines in the list such as
add
,get
andremove
. -
Cons: Relatively slow when searching through the list to find a medicine.
-
-
Alternative: Stores the medicines in a hash map.
-
Pros: Quick reference for object given the medicine name or serial number.
-
Cons: Cumbersome and error-prone when there is a need to change the hash map into a list to manipulate the medicines.
-